Delete a component in Angular

To delete a component in Angular, you need to:
Remove the import line reference from Angular app.module.ts file.
Remove the component declaration from @NgModule declaration array in app.module.ts file.
Manually delete the component folder from Angular project.
Delete all the references of component manually from the Angular project.
Note: Angular CLI does not currently support a command to delete a component, so you need to do it manually.
Here are the steps in more detail:
- Open the
src/app/app.module.ts
file. - Find the import line for the component you want to delete.
- Delete the import line.
- Find the component declaration in the
@NgModule
declaration array. - Delete the component declaration.
- Save the file.
- Open the folder containing the component you want to delete.
- Delete the folder.
- Search the project for any other references to the component and delete them.
Once you have completed these steps, the component will be removed from your Angular project.
Here is an example of how to delete a component called MyComponent
:
// Remove the import line reference.
import { MyComponent } from './my-component/my-component.component';
// Remove the component declaration from @NgModule declaration array.
@NgModule({
imports: [...],
declarations: [MyComponent],
providers: [...],
bootstrap: [AppComponent]
})
export class AppModule {}
// Delete the component folder.
src/app/my-component/my-component.component.html
src/app/my-component/my-component.component.css
src/app/my-component/my-component.component.ts
// Delete any other references to the component in the project.
Once you have completed these steps, the MyComponent
component will be removed from your Angular project.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Create Angular 17 Project Building a Password Strength Meter in Angular 17 Angular 17 Password Show/Hide with Eye Icon Angular 17 tutoriel Angular Select Change Event Angular iframe Angular FormArray setValue() and patchValue() Angular Find Substring in String Send File to API in Angular 17 EventEmitter Parent to Child Communication Create an Angular Material button with an icon and text Input change event in Angular 17 Find an element by ID in Angular 17 Find an element by ID from another component in Angular 17 Find duplicate objects in an array in JavaScript and Angular What is new with Angular 17 Style binding to text-decoration in Angular Remove an item from an array in Angular Remove a component in Angular Delete a component in Angular Use TypeScript enums in Angular templates Set the value of an individual reactive form fields Signal-based components in Angular 17 Angular libraries for Markdown: A comprehensive guide Angular libraries for cookies: A comprehensive guide Build an Angular 14 CRUD Example & Tutorial Angular 9 Components: Input and Output Angular 13 selectors Picture-in-Picture with JavaScript and Angular 10 Jasmine Unit Testing for Angular 12 Angular 9 Tutorial By Example: REST CRUD APIs & HTTP GET Requests with HttpClient Angular 10/9 Elements Tutorial by Example: Building Web Components Angular 10/9 Router Tutorial: Learn Routing & Navigation by Example Angular 10/9 Router CanActivate Guards and UrlTree Parsed Routes Angular 10/9 JWT Authentication Tutorial with Example Style Angular 10/9 Components with CSS and ngStyle/ngClass Directives Upload Images In TypeScript/Node & Angular 9/Ionic 5: Working with Imports, Decorators, Async/Await and FormData Angular 9/Ionic 5 Chat App: Unsubscribe from RxJS Subjects, OnDestroy/OnInit and ChangeDetectorRef Adding UI Guards, Auto-Scrolling, Auth State, Typing Indicators and File Attachments with FileReader to your Angular 9/Ionic 5 Chat App Private Chat Rooms in Angular 9/Ionic 5: Working with TypeScript Strings, Arrays, Promises, and RxJS Behavior/Replay Subjects Building a Chat App with TypeScript/Node.js, Ionic 5/Angular 9 & PubNub/Chatkit Chat Read Cursors with Angular 9/Ionic 5 Chat App: Working with TypeScript Variables/Methods & Textarea Keydown/Focusin Events Add JWT REST API Authentication to Your Node.js/TypeScript Backend with TypeORM and SQLite3 Database Building Chat App Frontend UI with JWT Auth Using Ionic 5/Angular 9 Install Angular 10 CLI with NPM and Create a New Example App with Routing Styling An Angular 10 Example App with Bootstrap 4 Navbar, Jumbotron, Tables, Forms and Cards Integrate Bootstrap 4/jQuery with Angular 10 and Styling the UI With Navbar and Table CSS Classes Angular 10/9 Tutorial and Example: Build your First Angular App Angular 9/8 ngIf Tutorial & Example Angular 10 New Features Create New Angular 9 Workspace and Application: Using Build and Serve Angular 10 Release Date: Angular 10 Will Focus on Ivy Artifacts and Libraries Support HTML5 Download Attribute with TypeScript and Angular 9 Angular 9.1+ Local Direction Query API: getLocaleDirection Example Angular 9.1 displayBlock CLI Component Generator Option by Example Angular 15 Basics Tutorial by Example Angular 9/8 ngFor Directive: Render Arrays with ngFor by Example Responsive Image Breakpoints Example with CDK's BreakpointObserver in Angular 9/8 Angular 9/8 DOM Queries: ViewChild and ViewChildren Example The Angular 9/8 Router: Route Parameters with Snapshot and ParamMap by Example Angular 9/8 Nested Routing and Child Routes by Example Angular 9 Examples: 2 Ways To Display A Component (Selector & Router) Angular 9/8 Tutorial: Http POST to Node/Express.js Example Angular 9/8 Feature and Root Modules by Example Angular 9/8 with PHP: Consuming a RESTful CRUD API with HttpClient and Forms Angular 9/8 with PHP and MySQL Database: REST CRUD Example & Tutorial Unit Testing Angular 9/8 Apps Tutorial with Jasmine & Karma by Example Angular 9 Web Components: Custom Elements & Shadow DOM Angular 9 Renderer2 with Directives Tutorial by Example Build Progressive Web Apps (PWA) with Angular 9/8 Tutorial and Example Angular 9 Internationalization/Localization with ngx-translate Tutorial and Example Create Angular 9 Calendar with ngx-bootstrap datepicker Example and Tutorial Multiple File Upload with Angular 9 FormData and PHP by Example Angular 9/8 Reactive Forms with Validation Tutorial by Example Angular 9/8 Template Forms Tutorial: Example Authentication Form (ngModel/ngForm/ngSubmit) Angular 9/8 JAMStack By Example Angular HttpClient v9/8 — Building a Service for Sending API Calls and Fetching Data Styling An Angular 9/8/7 Example App with Bootstrap 4 Navbar, Jumbotron, Tables, Forms and CardsHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
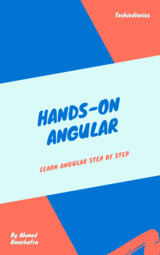