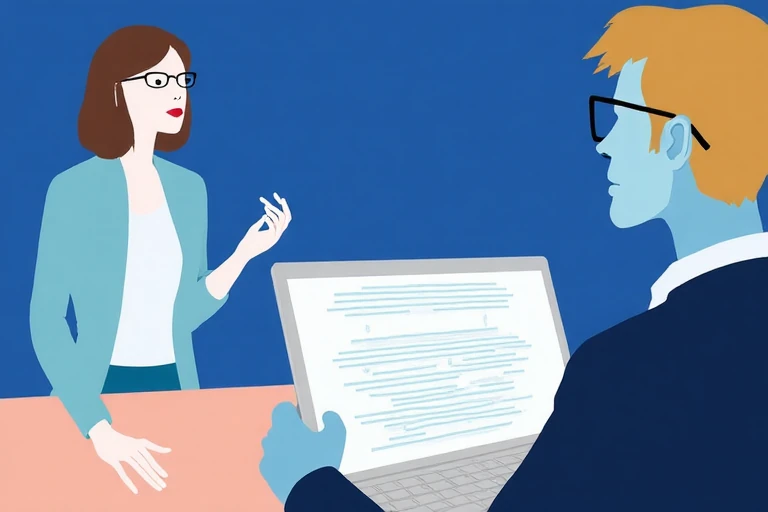
Angular 19 Integration with Firebase: Auth and Database
Angular's integration with Firebase continues to provide developers with powerful tools for building robust web applications. This article is about the current state of Angular 19 and Firebase integration, highlighting setup procedures, authentication methods, database operations with Firestore, and the benefits of this powerful stack.
Angular is a TypeScript-based open-source framework developed for building single-page applications with dynamic content loading and two-way data binding. It offers object-oriented features and supports efficient property and event binding techniques through its command-line interface for easy project management.
Firebase, on the other hand, is a Backend-as-a-Service (BaaS) provided by Google that eliminates the need for extensive backend development. It offers multiple services including authentication, real-time database, cloud storage, hosting, and real-time data synchronization with automatic update notifications. The use of Angular's frontend capabilities with Firebase's backend services creates a powerful full-stack development environment.
Setting Up Angular 19 with Firebase
Installation and Configuration
Setting up a new Angular 19 project with Firebase requires several steps, starting with creating an Angular application and adding the necessary Firebase packages:
ng new angular-firebase-app
cd angular-firebase-app
npm install firebase @angular/fire
The @angular/fire
package serves as an Angular wrapper for Firebase, providing a more natural developer experience by conforming to Angular conventions. After installation, you need to import the relevant modules into your application:
import { provideFirebaseApp, initializeApp } from '@angular/fire/app';
import { getFirestore, provideFirestore } from '@angular/fire/firestore';
export const appConfig: ApplicationConfig = {
providers: [
provideFirebaseApp(() =>; initializeApp({ /* Your Firebase config */ })),
provideFirestore(() =>; getFirestore()),
// Additional Firebase services as needed
],
};
This setup initializes the Firebase application using configuration keys obtained from the Firebase console. The @angular/fire
package offers several benefits including dependency injection, Zone.js wrappers for proper functionality, Observable-based patterns, and NgRx-friendly APIs.
Firebase Authentication with Angular
Implementing Authentication
Firebase provides multiple authentication methods, with email/password being one of the most common. To implement authentication:
- Configure authentication in the Firebase console
- Import authentication modules in your Angular application
- Create services to handle login, registration, and authentication state ```typescript import { inject } from '@angular/core'; import { Auth, signInWithEmailAndPassword } from '@angular/fire/auth';
export class AuthService { private auth = inject(Auth);
login(email: string, password: string) { return signInWithEmailAndPassword(this.auth, email, password); } } ```
Authentication with Firebase in Angular applications provides several built-in features including router guards that protect routes based on authentication status and user-friendly authentication flows.
Router Guards
Angular Firebase integrates seamlessly with Angular's routing system through built-in router guards:
import { canActivate, redirectUnauthorizedTo } from '@angular/fire/auth-guard';
const routes: Routes = [
{
path: 'profile',
component: ProfileComponent,
...canActivate(() => redirectUnauthorizedTo(['login']))
}
];
This setup redirects unauthorized users to the login page when they attempt to access protected routes.
Firestore Database Operations
Understanding Collections and Documents
Firebase Firestore uses a collection-document structure where collections are analogous to arrays in JavaScript and documents are similar to objects. This structure follows a pattern of collection-document-collection-document nesting.
CRUD Operations
Creating Data
Data creation in Firestore can be done using either add()
or set()
methods:
import { Firestore, collection, addDoc, setDoc, doc } from '@angular/fire/firestore';
// Using collection reference and add()
const userCollection = collection(this.firestore, 'users');
addDoc(userCollection, { name: 'John', age: 30 });
// Using document reference and set()
const userDocRef = doc(this.firestore, 'users', 'user123');
setDoc(userDocRef, { name: 'John', age: 30 });
The add()
method generates a random ID, while set()
allows you to specify the document ID.
Reading Data
Data retrieval can be implemented using various methods:
import { Firestore, collectionData, collection } from '@angular/fire/firestore';
import { Observable } from 'rxjs';
interface User {
name: string;
age: number;
}
// Getting a collection as an observable
const usersCollection = collection(this.firestore, 'users');
const users$: Observable<User[]> = collectionData<User>(usersCollection);
This approach provides an Observable that emits the latest data whenever changes occur in the database.
Updating and Deleting Data
Updates and deletions follow a similar pattern:
import { doc, updateDoc, deleteDoc } from '@angular/fire/firestore';
// Update a document
const userDocRef = doc(this.firestore, 'users', 'user123');
updateDoc(userDocRef, { age: 31 });
// Delete a document
deleteDoc(userDocRef);
Real-time Updates
One of Firebase's most powerful features is real-time data synchronization. Using Observables, Angular components can react to database changes:
import { AsyncPipe } from '@angular/common';
import { inject } from '@angular/core';
import { Firestore, collectionData, collection } from '@angular/fire/firestore';
@Component({
selector: 'app-user-list',
template: `
<ul>
@for (user of (users$ | async); track user) {
<li> - </li>
}
</ul>
`,
imports: [AsyncPipe]
})
export class UserListComponent {
firestore = inject(Firestore);
usersCollection = collection(this.firestore, 'users');
users$ = collectionData<User>(this.usersCollection);
}
This component automatically updates the UI whenever the database changes, without requiring manual refresh operations.
Advanced Features of AngularFire
Lazy Loading
AngularFire dynamically imports much of Firebase, reducing the initial load time of applications. This is particularly important for performance optimization in larger applications.
Deploy Schematics
The package provides schematics that simplify deployment to Firebase Hosting:
ng add @angular/fire
ng deploy
This allows deployment with a single command, streamlining the publishing process.
Google Analytics Integration
AngularFire offers zero-effort Angular Router awareness in Google Analytics, making it easy to track user navigation patterns:
import { provideAnalytics, getAnalytics } from '@angular/fire/analytics';
export const appConfig: ApplicationConfig = {
providers: [
// Other providers
provideAnalytics(() => getAnalytics()),
],
};
This integration automatically tracks route changes and sends them to Google Analytics.
Conclusion
Angular 19's integration with Firebase provides a robust framework for developing modern web applications with minimal backend configuration. The stack leverages Angular's powerful frontend capabilities with Firebase's comprehensive backend services to enable rapid development of feature-rich applications.
The Observable-based approach harmonizes perfectly with Angular's reactive programming model, while features like automatic data synchronization, authentication services, and deployment tooling streamline the development workflow. As both platforms continue to evolve, their integration represents a powerful solution for building scalable, real-time web applications with reduced development time and complexity.
For developers looking to build modern web applications quickly without extensive backend infrastructure, the Angular-Firebase stack remains one of the most efficient and feature-complete options available in the web development ecosystem.
Date: