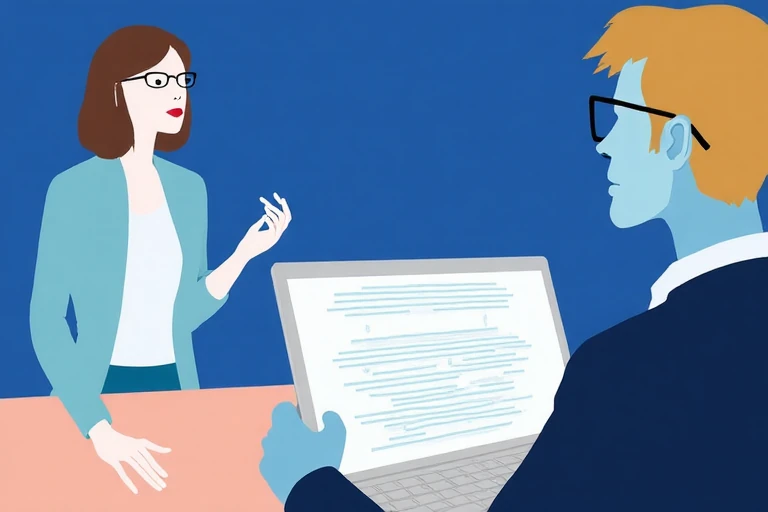
Use provideAppInitializer in Angular 19: APP_INITIALIZER is Deprecated
Angular 19 introduces significant changes to application initialization with the new provideAppInitializer function, which replaces the previously used and deprecated APP_INITIALIZER injection token. This post examines both approaches, their implementation differences, and migration strategies for developers upgrading to Angular 19.
What is APP_INITIALIZER in Angular
APP_INITIALIZER is an Angular injection token designed to execute one or more functions during application initialization, before the application is fully bootstrapped. This powerful feature allows developers to perform critical setup tasks that must complete before the application becomes available to users.
In Angular's initialization process, the framework searches for all providers associated with the APP_INITIALIZER token and executes their functions. These functions typically return either a Promise or an Observable, and Angular waits for all these to resolve before proceeding with application bootstrapping^1. This ensures that all necessary initialization steps are completed before the application is ready for user interaction.
The primary purpose of APP_INITIALIZER is to handle tasks such as loading configuration settings from servers, initializing services, or prefetching critical data^1. By completing these tasks during initialization, developers can ensure their applications start with all required resources and settings in place.
A basic implementation of APP_INITIALIZER in pre-Angular 19 applications looks like this:
import { APP_INITIALIZER, ApplicationConfig } from '@angular/core';
export const appConfig: ApplicationConfig = {
providers: [
{
provide: APP_INITIALIZER,
useFactory: initializeApp,
multi: true
}
]
};
export function initializeApp() {
return (): Promise<void> => {
return new Promise<void>((resolve) => {
console.log('Initialization complete');
setTimeout(() => {
resolve();
}, 5000); // Simulate a 5-second initialization task
});
};
}
In this example, the initializeApp
function simulates an asynchronous task that resolves after five seconds^1. The multi: true
property is crucial as it allows multiple initialization functions to be registered under the same token, which is essential for complex applications requiring multiple initialization tasks^1.
Angular 19's provideAppInitializer
With Angular 19, the framework introduced a new function called provideAppInitializer
that replaces the older APP_INITIALIZER token. This change aligns with Angular's ongoing transition toward a more functional approach to configuration and dependency injection.
The APP_INITIALIZER token has been deprecated in Angular 19, and developers are encouraged to migrate to the new provideAppInitializer
function^2. This function serves the same purpose as the previous token but with a more streamlined syntax that leverages Angular's dependency injection system more effectively.
The key difference between APP_INITIALIZER and provideAppInitializer is in how they're implemented. While the former required verbose configuration with provide
, useFactory
, and deps
properties, the new function simplifies this through a more direct approach that uses Angular's inject function within the initialization code^2.
The new syntax removes the need for explicitly defining dependencies through the deps
array, instead allowing developers to use the inject
function directly within their initializer function. This results in cleaner, more maintainable code that's easier to understand and debug.
Migrating from APP_INITIALIZER to provideAppInitializer
Migrating from APP_INITIALIZER to provideAppInitializer requires understanding the syntactical differences between the two approaches. Here's a step-by-step guide on how to migrate an existing initializer to the new pattern.
Before (using APP_INITIALIZER):
// Old approach
{
provide: APP_INITIALIZER,
useFactory: configFactory,
multi: true,
deps: [ConfigService]
}
// where configFactory is defined as:
export const configFactory = (config: ConfigService) => () => {
return config.loadAppConfig();
};
After (using provideAppInitializer):
// New approach
provideAppInitializer(() => {
const initializerFn = (configFactory)(inject(ConfigService));
return initializerFn();
})
// Or more concisely:
provideAppInitializer(() => {
const config = inject(ConfigService);
return config.loadAppConfig();
})
The new approach eliminates the need for the factory pattern where dependencies were explicitly defined. Instead, it uses Angular's inject
function to directly access the required services within the initializer function^2.
This migration follows the same pattern for other initialization tokens as well. For example, when migrating from ENVIRONMENT_INITIALIZER to provideEnvironmentInitializer, the same principles apply:
// Before
{
provide: ENVIRONMENT_INITIALIZER,
multi: true,
useValue() {
console.log('environment');
}
}
// After
provideEnvironmentInitializer(() => {
console.log('environment');
})
The simplified syntax makes the code more readable and maintainable while preserving the same functionality^2.
Practical Use Cases for Initializers
Initializers in Angular serve several practical purposes that significantly enhance application robustness and user experience. Let's explore some common use cases where APP_INITIALIZER or provideAppInitializer are particularly valuable.
Loading Configuration Settings from a Server
One of the most common use cases is fetching configuration data from a remote server before the application starts. This ensures that environment-specific settings or feature toggles are correctly loaded, influencing how the application behaves^1.
// Using provideAppInitializer
provideAppInitializer(() => {
const configService = inject(ConfigService);
return configService.get().pipe(
tap(config => {
localStorage.setItem('config', JSON.stringify(config));
})
);
})
This approach allows the application to have all necessary configuration parameters available before rendering any components, ensuring consistent behavior across the application^1.
Initializing Essential Services
Another important use case involves initializing critical services that must be ready before the application starts. This might include authentication services, logging frameworks, or other core infrastructure components^4.
provideAppInitializer(() => {
const authService = inject(AuthService);
return authService.initialize().pipe(
catchError(error => {
console.error('Failed to initialize auth service', error);
return of(null);
})
);
})
This ensures that essential services are properly initialized and ready to use when components begin rendering, preventing potential runtime errors or inconsistent states.
Detecting and Responding to System Events
Initializers can also be used to set up event listeners that need to be active throughout the application's lifecycle, such as router events^2:
provideEnvironmentInitializer(() => {
const router = inject(Router);
router.events.pipe(
filter(event => event instanceof Scroll)
).subscribe({
next: (e: Scroll) => {
console.log(e.position);
}
});
})
This pattern allows developers to centralize global event handling and ensure it's established before user interaction begins^2.
Potential Issues and Solutions
While migrating to provideAppInitializer is generally straightforward, some developers have reported issues when upgrading to Angular 19, particularly regarding the execution order of initialization functions.
Injection Token Hierarchy Issue
A notable issue reported by some developers involves a change in the execution order between APPINITIALIZER and InjectionTokens. In Angular 17, the APPINITIALIZER token was executed before other InjectionTokens were built, allowing developers to reference data in InjectionTokens that was retrieved within APP_INITIALIZER^3.
However, in Angular 19, some developers have observed that InjectionTokens are created before provideAppInitializer is executed, which can lead to undefined values if those tokens depend on data that should be retrieved during initialization^3.
According to Angular developers responding to this issue, provideAppInitializer is just a wrapper around APP_INITIALIZER and should behave the same way. If developers are experiencing differences in behavior, it might be due to specific implementation details or other changes in their application structure^3.
Solutions and Workarounds
If you encounter order-of-execution issues when migrating to provideAppInitializer, consider these approaches:
- Refactor your initialization strategy: Consider moving the initialization logic that sets up required data for other tokens into a separate service that runs earlier in the bootstrap process.
- Use dynamic providers: Instead of relying on static InjectionToken values that are defined at application startup, consider using factory providers that can retrieve the necessary values at the time they're needed.
- Report the issue: If you can reproduce a clear difference in behavior between APP_INITIALIZER and provideAppInitializer with a minimal example, reporting it to the Angular team might help identify if there's an actual regression in the framework.
Conclusion
Angular 19's introduction of provideAppInitializer represents a significant step in the framework's evolution toward more functional and straightforward APIs. The new approach simplifies initialization code and aligns with Angular's modern dependency injection system while maintaining the same core functionality.
For developers upgrading to Angular 19, migrating from APP_INITIALIZER to provideAppInitializer is generally straightforward, with the main changes being syntactical rather than conceptual. The new approach offers cleaner code with direct injection of dependencies, eliminating the need for explicit factory functions and dependency arrays.
While some developers have reported potential issues with the order of execution in the new implementation, most migrations should proceed smoothly. If you encounter specific problems, consider refactoring your initialization strategy or reporting reproducible issues to the Angular team.
As Angular continues to evolve, embracing these new patterns will help ensure your applications remain maintainable and aligned with current best practices in the Angular ecosystem.
Date: