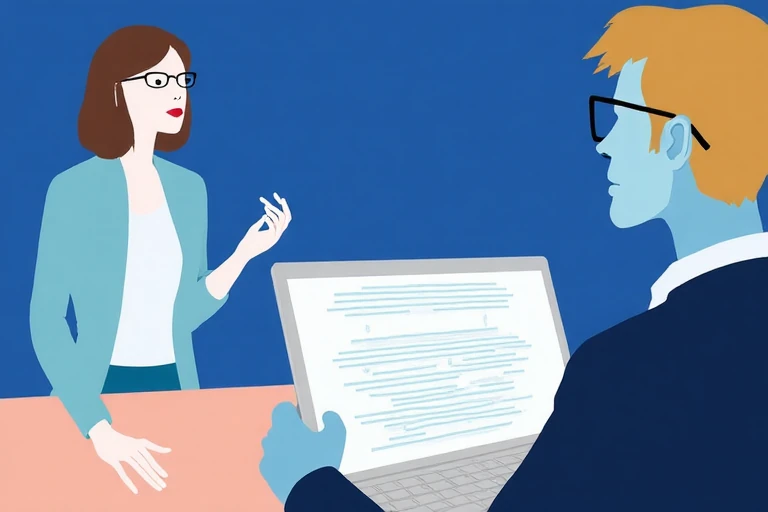
Introduction to React 19: What's New
In this series of articles, we'll present you with a detailed deep dive into React 19—focusing on all stable features, and including experimental ones, across both the core library and major ecosystem tools. we’ll also provide you with the insights from the React team and broader community, with summaries and examples designed for frontend developers.
We’ll explore what’s changed in React 19, organized into categories most relevant to frontend developers, with clear explanations, code examples, and insights from the React team and community.
React 19: What’s New
React 19 marks a significant update two years after React 18, introducing new features and improvements aimed at making React apps faster, more ergonomic, and easier to maintain. This overview dives into the stable release features as well as notable experimental additions, spanning the React core (react
, react-dom
) and key libraries like React Router, Redux, and Next.js.
(Note: React 19 went stable in late 2024, following an 18.3 release that backported warnings for deprecated APIs (React 19 Upgrade Guide – React). The upgrade is designed to be smooth for most apps, but it does involve some breaking changes which we’ll cover with guidance.)
Rendering & Performance Enhancements
React 19 brings multiple updates under the hood and in the API to improve rendering performance, resource loading, and hydration:
Faster Suspense Fallbacks: When a component “suspends” (e.g. waiting for data), React 19 now immediately shows the nearest
<Suspense>
fallback UI instead of rendering sibling components first (React 19 Upgrade Guide – React) (React 19 Upgrade Guide – React). This means your loading spinners or placeholder UIs appear sooner, improving perceived performance. After showing the fallback, React pre-warms the suspended data in the background so that by the time it’s ready, the rest of the UI can update smoothly (React 19 Upgrade Guide – React) (React 19 Upgrade Guide – React). In short, Suspense boundaries are more responsive, displaying fallback content faster while still preparing deferred data in parallel.Automatic Resource Preloading: React 19 introduces built-in APIs for preloading resources to optimize page load. New functions like
prefetchDNS
,preconnect
,preload
,preinit
, and their module-specific variants (preloadModule
,preinitModule
) let you hint to the browser to load resources early (What’s new in React 19 - Vercel) (What’s new in React 19 - Vercel). For example:import { prefetchDNS, preconnect, preload, preinit } from 'react-dom'; function App() { // Prepare connections and resources early prefetchDNS("https://api.example.com"); preconnect("https://api.example.com"); preload("/fonts/myfont.woff", { as: "font" }); preinit("/scripts/analytics.js", { as: "script" }); // ...normal component code }
This might output links in the HTML
<head>
like:<link rel="prefetch-dns" href="https://api.example.com"> <link rel="preconnect" href="https://api.example.com"> <link rel="preload" as="font" href="/fonts/myfont.woff"> <script async src="/scripts/analytics.js"></script> ``` ([React v19 – React](https://react.dev/blog/2024/12/05/react-19#:~:text=)) ([React v19 – React](https://react.dev/blog/2024/12/05/react-19#:~:text=%3Clink%20rel%3D)). These hints ensure critical resources (DNS, connections, fonts, scripts, styles) start loading *before* they are needed, speeding up both initial paints and subsequent navigations ([React v19 – React](https://react.dev/blog/2024/12/05/react-19#:~:text=)). Frontend developers can use these without a build step, and React will automatically avoid duplicating inserts (e.g. calling `preload` for the same URL twice will only inject one `<link>`).
Document Metadata Support: Managing
<head>
tags (like<title>
,<meta>
,<link>
) is simpler in React 19. You can now include these tags directly in your components’ render output, and React will hoist them to the document head during rendering (React v19 – React). For example, you can write a component that returns<title>{post.title}</title>
alongside your content, and React ensures the title (and other meta tags) end up in<head>
on both client and server render (React v19 – React) (React v19 – React). This replaces the need for external libraries like react-helmet in many cases (React v19 – React) (React v19 – React). It ensures metadata and SEO-critical tags work seamlessly with Client Components, streaming SSR, and React Server Components (RSC) out of the box. (Libraries may still add value for advanced cases like merging meta tags per route (React v19 – React).)Stylesheets and Scripts in Components: React 19 adds first-class support for including CSS and JS assets in your component tree:
- You can now render
<link rel="stylesheet">
and<style>
tags in components with a specialprecedence
attribute to manage their ordering (React v19 – React) (React v19 – React). By specifyingprecedence
, you tell React how to order styles relative to each other (e.g."high"
vs"default"
priority). React will automatically insert these style tags in the correct order in the DOM and ensure each stylesheet is loaded before showing content that depends on it (React v19 – React) (React v19 – React). It will also deduplicate stylesheets, so if two components both render the same stylesheet, it’s only loaded once (React v19 – React) (React v19 – React). This feature enables co-locating styles with components (for CSS or CSS-in-JS libraries that leverage it) without losing control over load timing. - For scripts, React now supports async scripts anywhere in the tree. In earlier React versions, including scripts in JSX was tricky and usually discouraged. In React 19, placing
<script async src="...">
in a component is allowed, and React will ensure such scripts are loaded and executed only once (even if rendered multiple times) (React v19 – React) (React v19 – React). On the server, async scripts get hoisted to the head (with appropriate priority behind CSS and fonts) so they don’t block rendering (React v19 – React). This is helpful for integrating third-party scripts or widgets directly in your component logic.
Why this matters: These improvements let you handle styles and scripts in a declarative way within React – you can think of them as part of your component’s render output, and React’s runtime will manage the details of injection order and duplicate prevention. It’s a step toward more seamless integration of external resources in React’s concurrent rendering model (React v19 – React) (React v19 – React), benefiting both CSR and SSR scenarios.
- You can now render
Hydration & Third-Party Scripts: Hydration (the process of attaching React to server-rendered HTML) is more robust in React 19, especially in the presence of third-party scripts or browser extensions. If a script (e.g., analytics) inserts extra DOM nodes into your HTML before React hydrates, React 18 would often error and re-render the whole app client-side. In React 19, unexpected nodes in
<head>
or<body>
are gracefully skipped during hydration (React v19 – React). React will ignore these extra elements rather than treating them as mismatches, preventing needless hydration failures. Furthermore, if a hydration error does force a full re-render, React now keeps third-party injected styles (like A/B test CSS or extension-added CSS) in place instead of wiping them out (React v19 – React) (React v19 – React). This means better resiliency when combining React with outside scripts – your app is less likely to “tear” or flicker due to hydration issues.Optimized Strict Mode (Dev Only): Though not affecting production directly, it’s worth noting React 19 made some Strict Mode dev-time behaviors less disruptive. For instance, in React 18, Strict Mode intentionally double-invoked certain functions (like component render and effects) to help catch bugs. This double-render also reset
useMemo
anduseCallback
values, which sometimes led to false positives or confusing logs. In React 19, during the Strict Mode double-render, memoized values from the first render are reused in the second render (React 19 Upgrade Guide – React). This means if your component is Strict Mode compliant, you shouldn’t see any difference, but if you were accidentally relying on side effects in those hooks, the behavior is closer to production now. It’s a minor improvement that reduces noise while still surfacing real issues (e.g., ref cleanup is still double-invoked to catch issues with mount/unmount logic (React 19 Upgrade Guide – React)).Other Performance Tweaks: React 19 removes some legacy baggage which can slightly improve bundle size and speed. Notably, UMD builds are no longer published (React 19 Upgrade Guide – React) (React 19 Upgrade Guide – React) – React now encourages using ESM bundles (e.g. via
<script type="module">
from a CDN) for those loading React via script tag. Also, internal optimizations like no longer rethrowing errors during render (discussed later) cut down unnecessary work. While these changes aren’t features you “use” in code, they contribute to React’s overall efficiency and maintainability.
Date: