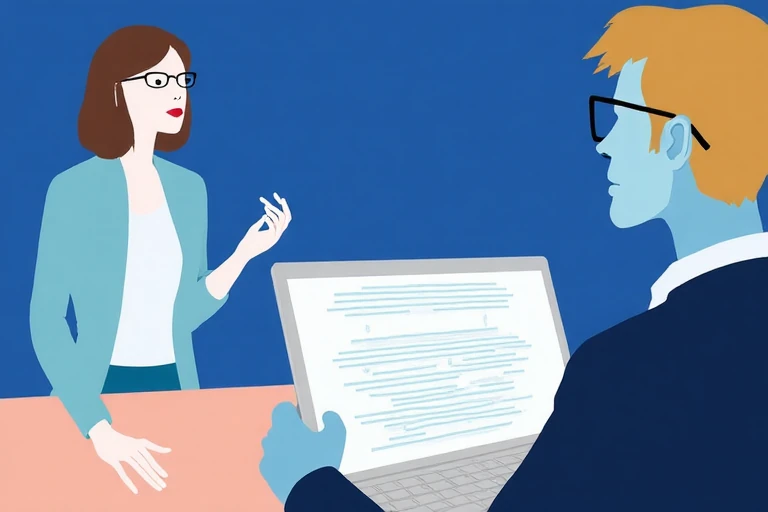
How can I pass parameters to an Angular 19 click event
To pass parameters to an Angular 19 click event, you can use the (click)
binding syntax and include the parameters directly within the method call in your template. Here are some examples:
Passing a Single Parameter
You can pass a single parameter to the event handler method like this:
<button (click)="handleClick('parameterValue')">Click me</button>;
In your component:
handleClick(param: string): void {
console.log('Button clicked with parameter:', param);
}
When the button is clicked, the handleClick
method will log the passed parameter value^1.
Passing Multiple Parameters
To pass multiple parameters, include them in the method call:
<button (click)="handleClick('param1', 'param2')">Click me</button>
In your component:
handleClick(param1: string, param2: string): void {
console.log(`Button clicked with parameters: ${param1}, ${param2}`);
}
This approach allows you to customize the behavior of your handler based on multiple inputs^5.
Combining Parameters with Event Data
You can also pass both custom parameters and the $event
object:
<button (click)="handleClick($event, 'customParam')">Click me</button>
In your component:
handleClick(event: MouseEvent, customParam: string): void {
console.log('Event:', event);
console.log('Custom parameter:', customParam);
}
This is useful when you need both event details (e.g., mouse coordinates) and additional context^2.
Dynamic Parameter Passing
You can use Angular's property binding to dynamically pass parameters:
<button (click)="handleClick(dynamicParam)">Click me</button>
Where dynamicParam
is a variable defined in your component:
dynamicParam = 'Dynamic Value';
handleClick(param: string): void {
console.log('Dynamic parameter:', param);
}
These techniques provide flexibility in customizing click events and handling user interactions effectively.
Date: