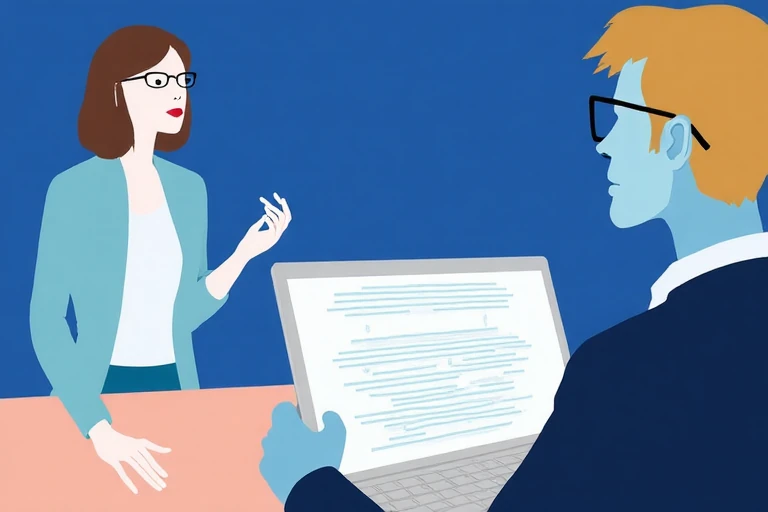
Introduction to React 19: DevTools & Debugging Improvements
While many of React 19’s changes are about new capabilities, the release also includes several quality-of-life improvements for developers debugging their apps:
Better Error Logs: React 19 significantly cleaned up the console output for runtime errors. In React 18, if an error was thrown during render and caught by an Error Boundary, you often saw a confusing trio of logs: one error, one rethrown error, and a logged component stack (React 19 Upgrade Guide – React) (React 19 Upgrade Guide – React). React 19 stops rethrowing caught errors (which previously led to duplicate logs) (React 19 Upgrade Guide – React) (React 19 Upgrade Guide – React). Now, if an Error Boundary catches an error, React will log just one consolidated error message to the console, including the component stack and a note that React will recover using the boundary (React v19 – React) (React v19 – React). Similarly, uncaught errors (no Error Boundary) will be reported via
window.reportError
once instead of twice (React 19 Upgrade Guide – React). The result is a much cleaner dev console – you see the real issue once, with all relevant info. This addresses a long-standing annoyance where developers would see double errors or “Already attempted to recover” messages that didn’t clearly signal if it was one error or two different errors.New Error Handling Hooks: To give developers more control, two new callbacks can be provided when creating a React root:
onCaughtError
andonUncaughtError
(React v19 – React). These complement the existingonRecoverableError
. You would use these when callingcreateRoot(container, { ... })
. For example:createRoot(domNode, { onCaughtError(error, errorInfo) { // Called when an ErrorBoundary catches an error logErrorToService(error, errorInfo); }, onUncaughtError(error, errorInfo) { // Called when an error is uncaught (boundary missing) reportCritical(error); }, onRecoverableError(error, errorInfo) { // (same as React 18) - for non-fatal errors like hydration mismatches } });
These hooks let you intercept errors globally in a single place rather than sprinkling logic in components. For instance, you might use
onCaughtError
to track frontend exceptions that were gracefully handled, for monitoring purposes (React v19 – React). Prior to this, if you wanted to know about errors caught by boundaries, you had to wrap or patch the console or use the legacy error logging API on boundaries. This new approach is cleaner.Hydration Mismatch Warnings: If you’ve done server rendering, you might be familiar with the dreaded “Warning: Text content did not match. Server: X Client: Y” messages. React 19 improves these messages. Instead of logging repetitive warnings and a generic error, it now logs a single detailed error explaining that hydration failed and why (React v19 – React) (React v19 – React). It even provides a diff-like output showing what was expected vs received in the HTML, which is super helpful for debugging mismatches (React v19 – React) (React v19 – React). The message also lists common causes (like using
Math.random()
in server output or differing locale formatting) to guide developers (React v19 – React). This is a welcome change – hydration issues can be tricky to trace, and a clear error can save a lot of time. The error now also includes a link to a React doc page on hydration mismatches for further guidance (React v19 – React).DevTools Support: React Developer Tools was updated around the time of React 19 to support its new features. For example, the React DevTools Profiler is aware of the React Compiler’s automatic memoizations (if in use) and the new hooks like
useOptimistic
. The components tree will also show the new<Context>
usage properly. If you inspect a component that uses a Server Component or an Action, DevTools will identify it (though inspecting server components from the browser has inherent limitations since they aren’t running in the browser). There was also work to show why a component re-rendered, which ties in nicely with the compiler – if something was skipped due to memoization, DevTools can indicate that.Additionally, Next.js 15 introduced an improved Fast Refresh and error overlay. While not strictly part of React 19, these ecosystem devtools were tuned to work well with it – e.g., if a Server Action throws an error, Next.js overlay can display it with stack trace, and React 19’s improved error logging makes that output clearer.
Strict Mode Warnings: Some development-only warnings were added or refined. For example, using the deprecated APIs (that we’ll mention in breaking changes) will log warnings in 18.3 and above, so by the time you’re on 19 you ideally have fixed them. React 19 also warns if you use the old JSX transform (pre-2020). The new JSX transform (which doesn’t require
import React
) is required now (React 19 Upgrade Guide – React) (React 19 Upgrade Guide – React) – if you somehow haven’t migrated, React 19 will console.warn and possibly fail to compile. This is because new features like theref
prop and some performance improvements rely on the new transform.DevTools Performance Highlight: One interesting community find was that React 19 nearly shipped a regression that almost made the internet slower, but it was caught and fixed before release. A user on Hacker News pointed out that a change in React 19’s approach to scheduling could have impacted common usage patterns – the React team addressed this during the RC phase. It shows their commitment to not regressing performance; they even delayed the release a bit to fine-tune things like that.
Conclusion
Overall, React 19’s developer experience is improved by surfacing clearer errors and giving more hooks to handle them. To quote a core team member, the goal was to remove noise and make real issues stand out: “we improved how errors are handled to reduce duplication by not re-throwing [errors during render]” (React 19 Upgrade Guide – React) (React 19 Upgrade Guide – React). The result is less time scratching your head at confusing logs and more actionable info when something does go wrong.
Date: