Angular 17 Password Show/Hide with Eye Icon

In modern web development, user experience plays a crucial role in determining the success of an application. One aspect of user experience involves providing intuitive features like password show/hide functionality. Angular 17, a popular JavaScript framework, offers a convenient way to implement this feature seamlessly. In this tutorial, we'll walk through the process of integrating a password show/hide toggle functionality with an eye icon using Angular 17.
Prerequisites:
- Basic knowledge of HTML, CSS, and JavaScript.
- Familiarity with Angular 17 framework and Angular 17 CLI.
Step 1: Set Up Your Angular 17 Project
If you haven't already installed Angular 17 CLI, you can do so by running the following command:
npm install -g @angular/cli
Create a new Angular project using Angular 17 CLI:
ng new angular-password-show-hide
cd angular-password-show-hide
Step 2: Create a New Angular 17 Component
Generate a new Angular 17 component to handle the password show/hide functionality:
ng generate component password-toggle
Step 3: Implement Password Toggle Functionality
Navigate to the newly created component file password-toggle.component.ts
and update it with the following code:
import { Component } from '@angular/core';
@Component({
selector: 'app-password-toggle',
templateUrl: './password-toggle.component.html',
styleUrls: ['./password-toggle.component.css']
})
export class PasswordToggleComponent {
hide = true;
toggleVisibility(): void {
this.hide = !this.hide;
}
}
Step 4: Create HTML Template
Navigate to password-toggle.component.html
and add the following HTML markup:
<div class="password-container">
<input type="" placeholder="Enter Password">
<button (click)="toggleVisibility()">
<i class="fa" [ngClass]="{'fa-eye': hide, 'fa-eye-slash': !hide}"></i>
</button>
</div>
Step 5: Style the Component (Optional)
Navigate to password-toggle.component.css
and add styles to customize the appearance of the password input and toggle button according to your design preferences.
Step 6: Include Font Awesome Icons (Optional)
If you're using Font Awesome icons, make sure to include the Font Awesome CSS file in your project's index.html
:
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.4/css/all.min.css">
Step 7: Add the Password Toggle Component to Your Application
Open app.component.html
and include the password toggle component:
<app-password-toggle></app-password-toggle>
Step 8: Run Your Angular 17 Application
Save all changes and run your Angular 17 application using the following command:
ng serve
Visit http://localhost:4200
in your web browser to see the password show/hide functionality in action.
Conclusion:
In this tutorial, you've learned how to implement the password show/hide feature with an eye icon using Angular 17. This feature enhances user experience by providing users with the option to toggle password visibility, thus improving the usability and accessibility of your web application. Experiment with different styling and customization options to tailor the feature according to your project requirements. Happy coding!
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Create Angular 17 Project Building a Password Strength Meter in Angular 17 Angular 17 Password Show/Hide with Eye Icon Angular 17 tutoriel Angular Select Change Event Angular iframe Angular FormArray setValue() and patchValue() Angular Find Substring in String Send File to API in Angular 17 EventEmitter Parent to Child Communication Create an Angular Material button with an icon and text Input change event in Angular 17 Find an element by ID in Angular 17 Find an element by ID from another component in Angular 17 Find duplicate objects in an array in JavaScript and Angular What is new with Angular 17 Style binding to text-decoration in Angular Remove an item from an array in Angular Remove a component in Angular Delete a component in Angular Use TypeScript enums in Angular templates Set the value of an individual reactive form fields Signal-based components in Angular 17 Angular libraries for Markdown: A comprehensive guide Angular libraries for cookies: A comprehensive guide Build an Angular 14 CRUD Example & Tutorial Angular 9 Components: Input and Output Angular 13 selectors Picture-in-Picture with JavaScript and Angular 10 Jasmine Unit Testing for Angular 12 Angular 9 Tutorial By Example: REST CRUD APIs & HTTP GET Requests with HttpClient Angular 10/9 Elements Tutorial by Example: Building Web Components Angular 10/9 Router Tutorial: Learn Routing & Navigation by Example Angular 10/9 Router CanActivate Guards and UrlTree Parsed Routes Angular 10/9 JWT Authentication Tutorial with Example Style Angular 10/9 Components with CSS and ngStyle/ngClass Directives Upload Images In TypeScript/Node & Angular 9/Ionic 5: Working with Imports, Decorators, Async/Await and FormData Angular 9/Ionic 5 Chat App: Unsubscribe from RxJS Subjects, OnDestroy/OnInit and ChangeDetectorRef Adding UI Guards, Auto-Scrolling, Auth State, Typing Indicators and File Attachments with FileReader to your Angular 9/Ionic 5 Chat App Private Chat Rooms in Angular 9/Ionic 5: Working with TypeScript Strings, Arrays, Promises, and RxJS Behavior/Replay Subjects Building a Chat App with TypeScript/Node.js, Ionic 5/Angular 9 & PubNub/Chatkit Chat Read Cursors with Angular 9/Ionic 5 Chat App: Working with TypeScript Variables/Methods & Textarea Keydown/Focusin Events Add JWT REST API Authentication to Your Node.js/TypeScript Backend with TypeORM and SQLite3 Database Building Chat App Frontend UI with JWT Auth Using Ionic 5/Angular 9 Install Angular 10 CLI with NPM and Create a New Example App with Routing Styling An Angular 10 Example App with Bootstrap 4 Navbar, Jumbotron, Tables, Forms and Cards Integrate Bootstrap 4/jQuery with Angular 10 and Styling the UI With Navbar and Table CSS Classes Angular 10/9 Tutorial and Example: Build your First Angular App Angular 9/8 ngIf Tutorial & Example Angular 10 New Features Create New Angular 9 Workspace and Application: Using Build and Serve Angular 10 Release Date: Angular 10 Will Focus on Ivy Artifacts and Libraries Support HTML5 Download Attribute with TypeScript and Angular 9 Angular 9.1+ Local Direction Query API: getLocaleDirection Example Angular 9.1 displayBlock CLI Component Generator Option by Example Angular 15 Basics Tutorial by Example Angular 9/8 ngFor Directive: Render Arrays with ngFor by Example Responsive Image Breakpoints Example with CDK's BreakpointObserver in Angular 9/8 Angular 9/8 DOM Queries: ViewChild and ViewChildren Example The Angular 9/8 Router: Route Parameters with Snapshot and ParamMap by Example Angular 9/8 Nested Routing and Child Routes by Example Angular 9 Examples: 2 Ways To Display A Component (Selector & Router) Angular 9/8 Tutorial: Http POST to Node/Express.js Example Angular 9/8 Feature and Root Modules by Example Angular 9/8 with PHP: Consuming a RESTful CRUD API with HttpClient and Forms Angular 9/8 with PHP and MySQL Database: REST CRUD Example & Tutorial Unit Testing Angular 9/8 Apps Tutorial with Jasmine & Karma by Example Angular 9 Web Components: Custom Elements & Shadow DOM Angular 9 Renderer2 with Directives Tutorial by Example Build Progressive Web Apps (PWA) with Angular 9/8 Tutorial and Example Angular 9 Internationalization/Localization with ngx-translate Tutorial and Example Create Angular 9 Calendar with ngx-bootstrap datepicker Example and Tutorial Multiple File Upload with Angular 9 FormData and PHP by Example Angular 9/8 Reactive Forms with Validation Tutorial by Example Angular 9/8 Template Forms Tutorial: Example Authentication Form (ngModel/ngForm/ngSubmit) Angular 9/8 JAMStack By Example Angular HttpClient v9/8 — Building a Service for Sending API Calls and Fetching Data Styling An Angular 9/8/7 Example App with Bootstrap 4 Navbar, Jumbotron, Tables, Forms and CardsHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
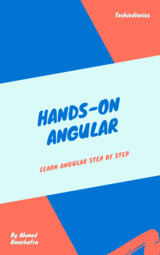