Angular 17 AfterRender & afterNextRender

Angular 16 introduces two novel lifecycle hooks: afterRender and afterNextRender. These hooks empower developers to execute code after a component has been rendered—be it the initial render (afterNextRender) or any subsequent render (afterRender).
This represents a substantial enhancement over existing lifecycle hooks, such as ngAfterViewInit, which trigger after a component has been initialized but before any DOM updates have occurred. This can pose challenges when working with the DOM in ngAfterViewInit, as one often needs to await DOM updates before interacting with them.
The afterRender and afterNextRender hooks, however, guarantee execution after DOM updates, rendering them ideal for DOM-related tasks like invoking third-party libraries or direct DOM manipulation.
Additionally, it's worth noting that these hooks do not run during server-side rendering (SSR), simplifying their use in SSR applications where you don't want your code to run twice—once on the server and again on the client.
Let's delve into an example showcasing the usage of the afterRender hook to invoke a third-party library:
import { Component, AfterViewInit } from '@angular/core';
import { MyThirdPartyLibrary } from 'my-third-party-library';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements AfterViewInit {
constructor(private myThirdPartyLibrary: MyThirdPartyLibrary) {}
ngAfterViewInit() {
// This code will not work, as the DOM has not been updated yet.
this.myThirdPartyLibrary.init();
}
afterRender() {
// This code will work, as the DOM has been updated.
this.myThirdPartyLibrary.init();
}
}
Benefits of Leveraging afterRender and afterNextRender Hooks:
Simplified DOM Interaction: These hooks ensure that the DOM is updated before your code executes, simplifying DOM-related tasks.
Enhanced SSR Compatibility: These hooks exclude SSR execution, making them well-suited for SSR applications without concerns about code duplication.
Improved Performance: Delaying code execution until after DOM updates can enhance your application's performance by minimizing unnecessary operations.
Conclusion
In conclusion, Angular 16's afterRender and afterNextRender hooks offer a potent avenue for engaging with the DOM. They streamline code development, boost performance, and facilitate interactions with the DOM, ushering in a new era of Angular development.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
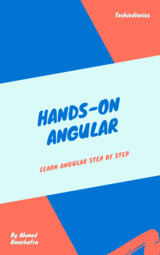