Angular Injection Context: What is it and how to use it

In the realm of reactive programming, an Observable is a pivotal concept. It serves as a push-based stream of data that encapsulates changes occurring over time. Observables offer a powerful mechanism for handling asynchronous events and data streams in a clean and structured manner. Let's embark on a journey to delve into the intricacies of Observables and related concepts.
Understanding Observables
Observables, as the name suggests, allow developers to observe and react to changes in data over time. They are particularly useful when dealing with asynchronous operations, such as HTTP requests, user interactions, or event-driven programming.
Key Characteristics of Observables:
Push-Based Data Streams: Observables follow a push-based model, meaning that they proactively emit data to Observers when new values are available. Observers don't need to repeatedly poll for updates; they simply subscribe and wait for notifications.
Lazy Execution: Observables are lazy, which means they do not start emitting data until an Observer subscribes to them. This lazy behavior ensures that resources are not wasted on data streams that are not actively observed.
Subject: The Special Observer-Observable Hybrid
A Subject is a remarkable extension of the Observable concept. Not only can it serve as an Observable, but it can also act as an Observer. This dual nature enables Subjects to subscribe to other Observables and relay their emitted values to their own set of Observers.
Key Characteristics of Subjects:
Observables and Observers: Subjects seamlessly play the roles of both Observable and Observer. They can emit values to their Observers while also subscribing to other Observables to capture external data.
Hot Observable: Subjects are considered "hot" Observables. This means they emit data regardless of when Observers subscribe. All values are broadcasted to all Observers, making them suitable for scenarios where data sharing is essential.
BehaviorSubject: A Specialized Subject for State Management
A BehaviorSubject is a specific type of Subject that offers a distinct feature: it stores and emits the latest emitted value to new Observers when they subscribe. This property makes BehaviorSubjects particularly valuable for managing shared state in Angular applications.
Key Characteristics of BehaviorSubjects:
Stateful Emissions: BehaviorSubjects maintain an internal state and emit the most recent value to new subscribers. This behavior ensures that new Observers receive the latest state, making them suitable for managing shared application state. Choosing the Right Tool for the Job Determining which tool to use—Observable, Subject, or BehaviorSubject—depends on your specific use case:
Observable: Employ Observables to represent data streams emitted over time, such as HTTP responses or user events. They are the foundation for handling asynchronous data.
Subject: Subjects are versatile and ideal for custom data streams that need to be observed and shared among multiple components. Use them for inter-component communication or custom event handling.
BehaviorSubject: When you need to manage shared state across various components in an Angular application, BehaviorSubjects shine. They ensure that all subscribers receive the current state upon subscription.
Practical Example: Using BehaviorSubject in Angular
Let's explore a practical scenario where a BehaviorSubject is employed to manage the state of a component:
import { Component, OnInit } from '@angular/core';
import { BehaviorSubject } from 'rxjs';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
private _selectedUser = new BehaviorSubject<string>('');
constructor() { }
ngOnInit() {
// Set the initial value of the BehaviorSubject.
this._selectedUser.next('John Doe');
}
// Subscribe to the BehaviorSubject to receive notifications of changes.
subscribeToSelectedUser() {
this._selectedUser.subscribe(user => {
// Perform actions with the selected user.
});
}
// Emit a new value to the BehaviorSubject.
setSelectedUser(user: string) {
this._selectedUser.next(user);
}
}
In this example, the _selectedUser BehaviorSubject maintains the currently selected user within the component. Any component requiring knowledge of the selected user can subscribe to the BehaviorSubject to receive timely updates.
Conclusion
Observables, Subjects, and BehaviorSubjects are foundational concepts in the world of RxJS and Angular. Understanding the distinctions between these constructs empowers developers to make informed decisions when handling asynchronous data and state management. Whether you're building small-scale applications or complex Angular projects, choosing the appropriate tool ensures efficient and maintainable code.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
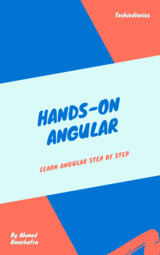