Dynamically loading Angular components

Angular offers a robust technique for dynamically loading components, enabling the creation of more flexible and responsive applications. This approach loads components on-demand, precisely when they are required, which can significantly enhance performance and reduce bundle size.
There are two primary methods to achieve dynamic component loading in Angular:
Using the ComponentFactoryResolver
The ComponentFactoryResolver is a service that facilitates the dynamic creation of component instances. To utilize it, begin by injecting it into your component. Subsequently, employ the resolveComponentFactory() method to obtain a factory for the target component.
Once you have the factory, utilize the createComponent() method to instantiate the component dynamically. Finally, you can insert the component into the DOM using the ViewContainerRef.
Below is an example of how to dynamically load a component using the ComponentFactoryResolver:
import { Component, ComponentFactoryResolver, ViewContainerRef, Type } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
})
export class AppComponent {
constructor(
private componentFactoryResolver: ComponentFactoryResolver,
private viewContainerRef: ViewContainerRef,
) {}
loadComponent(componentType: Type<any>) {
const factory = this.componentFactoryResolver.resolveComponentFactory(componentType);
const componentRef = factory.create(this.viewContainerRef);
this.viewContainerRef.insert(componentRef.hostView);
}
}
Using the RouterModule
The RouterModule can also be employed for dynamic component loading. To achieve this, leverage the loadChildren property of a route. The loadChildren property can be a function that returns a promise resolving to a module. The module must export the component you intend to load.
Here is an example of how to dynamically load a component using the RouterModule:
import { RouterModule, Routes } from '@angular/router';
const routes: Routes = [
{
path: 'my-component',
loadChildren: () => import('./my-component/my-component.module').then((m) => m.MyComponentModule),
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule],
})
export class AppRoutingModule {}
Use Cases for Dynamic Component Loading
The dynamic loading of components in Angular is a versatile approach with numerous use cases, including:
Lazy Loading: It can significantly enhance application performance by loading components only when they are required. This is particularly beneficial for large applications with numerous components.
Dynamic UI: Dynamic loading allows for the creation of more dynamic and responsive user interfaces. For example, you can load different components based on the user's role or preferences.
-Third-Party Components: Dynamic loading is an effective strategy for incorporating third-party components into your application, helping avoid bundling all third-party code into your application bundle.
Conclusion
Dynamically loading components in Angular is a potent technique that empowers developers to create more adaptable and responsive applications. It is relatively straightforward to learn and can be applied to a wide range of use cases, making it a valuable addition to your Angular development toolkit.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
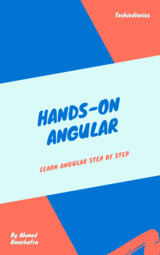