Angular Signals and HttpClient

Angular signals represent a groundbreaking development in the world of Angular. These reactive primitives promise to transform the way we develop Angular applications, offering a more streamlined and developer-friendly experience. Furthermore, Angular signals are poised to revolutionize the change detection mechanism, making it more efficient and intuitive.
The Power of Angular Signals
Angular signals bring forth a host of advantages for developers. One of the standout benefits is their seamless integration with the Angular HttpClient, a vital tool for making HTTP requests. While HttpClient is a potent resource, handling multiple asynchronous requests can be intricate. Angular signals simplify this process significantly.
Converting HttpClient Observables to Signals
To harness the benefits of Angular signals, we can convert HttpClient Observables to signals using the toSignal()
function. This function takes an Observable as input and returns a signal that emits the same values as the Observable. Consider the following code example:
import { HttpClient } from '@angular/common/http';
import { toSignal } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class UserService {
constructor(private http: HttpClient) {}
getUsers(): Signal<User[]> {
const observable = this.http.get<User[]>('/api/users');
return toSignal(observable);
}
}
Here, we've converted an Observable that retrieves a list of users into a signal. This transformation simplifies our code and enhances manageability.
Using Signals in Components
Signals can be utilized in components in a manner similar to Observables. You can bind to them using the async pipe and subscribe to them using the subscribe()
method. For instance, you can bind to a signal that returns a list of users in a component template like this:
<ul>
<li *ngFor="let user of users | async">
</li>
</ul>
This straightforward approach ensures that your UI remains synchronized with the data emitted by signals.
Creating Computed Signals
Another powerful feature of Angular signals is their ability to create computed values. Computed values are derived from other signals and are automatically updated whenever the underlying signals change. To create computed signals, you can use the computed()
function, which takes a function as input to calculate the value of the computed signal.
Here's an example of creating a computed signal that returns the total number of users in a list:
import { computed } from '@angular/core';
@Component({
selector: 'app-my-component',
templateUrl: './my-component.html',
styleUrls: ['./my-component.css']
})
export class MyComponent {
users: Signal<User[]> = toSignal(this.userService.getUsers());
totalUsers: Signal<number> = computed(() => this.users.value.length);
constructor(private userService: UserService) {}
}
With this setup, you can bind to the totalUsers
signal in your component template just like any other signal.
The Advantages of Angular Signals with HttpClient
The adoption of Angular signals alongside HttpClient yields numerous benefits, including:
Improved Performance Signals are more efficient than Observables because they don't need to subscribe to the underlying Observable. This efficiency can lead to significant performance improvements, especially in applications that make numerous HTTP requests.
Simplified Code Angular signals simplify the writing and maintenance of code that deals with asynchronous HTTP requests. Signals enable you to chain requests, manage multiple requests concurrently, and handle errors more gracefully.
Enhanced Developer Experience Working with asynchronous data becomes more intuitive with signals. They are easier to understand and reason about than Observables, which can be complex and challenging to debug.
Incorporating Angular signals into your Angular applications, particularly when working in conjunction with HttpClient, can lead to more efficient and maintainable code. If you're new to Angular signals, it's recommended to consult the official documentation and explore the wealth of tutorials and blog posts available online. Embracing Angular signals is a step toward a more efficient, developer-friendly, and robust Angular development journey.
- Author: Ahmed Bouchefra Follow @ahmedbouchefra
-
Date:
Related posts
Angular Signals & Forms Angular 16 Injecting Service without Constructor Angular @Input View Transitions API in angular 17 tutorial View Transitions API in angular 17 tutorial Dynamically loading Angular components Angular 17 AfterRender & afterNextRender Angular Standalone Component Routing Angular Standalone Components vs. Modules Angular 17 resolvers Angular 17 Error Handling: What's New and How to Implement It Angular Signals in templates Angular Signals and HttpClient Angular Signals CRUD step by step Angular Injection Context: What is it and how to use it Angular Injection Context: What is it and how to use it How to Avoid Duplicate HTTP Requests Angular 17 — Deferred Loading Using Defer Block Asynchronous pipes in Angular: A Deeper dive Top 5 Angular Carousel Components of 2023 Angular 17 Material Multi Select Dropdown with Search Angular 17: Enhanced Control Flow Simplified with Examples Angular 17 Material Autocomplete Multiselect Step-by-Step Angular 17 Material Autocomplete Get Selected Value Example Angular 17 Material Alert Message Step by Step A Step-by-Step Guide to Using RxJS combineLatestWith RxJS Buffer: An Effective Tool for Data Grouping and Filtering Angular 14+ standalone components Angular v17 tutorial Angular 17 CRUD Tutorial: Consume a CRUD REST API Upgrade to Angular 17 Angular 17 standalone component Add Bootstrap 5 to Angular 17 with example & tutorial Angular 17 due date and new features Angular 17 Signals ExplainedHands-on Angular eBook
Subscribe to our Angular newsletter and get our hands-on Angular book for free!
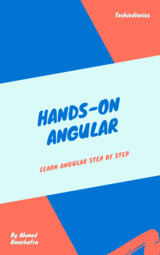